|
import QtQuick 2.15 |
|
import QtQuick.Templates 2.15 as T |
|
import QtQuick.Shapes 1.15 |
|
import QtGraphicalEffects 1.15 |
|
|
|
T.Dial { |
|
id: control |
|
implicitWidth: Math.max(implicitBackgroundWidth + leftInset + rightInset, |
|
implicitContentWidth + leftPadding + rightPadding) |
|
implicitHeight: Math.max(implicitBackgroundHeight + topInset + bottomInset, |
|
implicitContentHeight + topPadding + bottomPadding) |
|
|
|
component Gauge: Item { |
|
id: gauge |
|
width: 220 |
|
height: 220 |
|
property real sweepAngle: 0 |
|
Shape { |
|
anchors.fill: parent |
|
layer.enabled: true |
|
layer.samples: 8 |
|
ShapePath { |
|
strokeWidth: gauge.width * 0.20 |
|
strokeColor: '#fff' |
|
fillColor: 'transparent' |
|
capStyle: ShapePath.FlatCap |
|
strokeStyle: ShapePath.DashLine |
|
dashPattern: [10/gauge.width,10/gauge.width] |
|
|
|
PathAngleArc { |
|
id: arc |
|
centerX: gauge.width/2; centerY: centerX |
|
radiusX: centerX * 0.76; radiusY: radiusX |
|
startAngle: -90 - 149 |
|
sweepAngle: Math.max(0,gauge.sweepAngle) |
|
} |
|
} |
|
} |
|
} |
|
|
|
component ShadedGauge: RadialGradient { |
|
id: shadedGauge |
|
property real sweepAngle: 0 |
|
|
|
layer.enabled: true |
|
layer.effect: OpacityMask { |
|
maskSource: Gauge { |
|
width: shadedGauge.width |
|
height: shadedGauge.height |
|
sweepAngle: shadedGauge.sweepAngle |
|
} |
|
} |
|
} |
|
|
|
|
|
background: Item { |
|
implicitWidth: 184 |
|
implicitHeight: 184 |
|
|
|
ShadedGauge { |
|
sweepAngle: 298 |
|
width: parent.width |
|
height: parent.width |
|
gradient: Gradient { |
|
GradientStop { position: 0.300; color: "#234" } |
|
GradientStop { position: 0.350; color: "#000" } |
|
GradientStop { position: 0.355; color: "#234" } |
|
} |
|
} |
|
ShadedGauge { |
|
sweepAngle: 149 + control.angle * 1.07 |
|
width: parent.width |
|
height: parent.width |
|
gradient: Gradient { |
|
GradientStop { position: 0.300; color: "#fff" } |
|
GradientStop { position: 0.350; color: "#777" } |
|
GradientStop { position: 0.355; color: "#fff" } |
|
GradientStop { position: 0.460; color: "#fff" } |
|
GradientStop { position: 0.465; color: "#234" } |
|
GradientStop { position: 0.470; color: "#234" } |
|
GradientStop { position: 0.480; color: "#b83" } |
|
} |
|
} |
|
} |
|
|
|
handle: Item { |
|
width: control.width |
|
height: control.height |
|
rotation: control.angle * 1.07 |
|
|
|
Rectangle { |
|
y: 1; x: (parent.width - width)/2 |
|
width: 4; height: 4; radius: 1 |
|
smooth: true |
|
} |
|
|
|
Rectangle { |
|
y: 1; x: (parent.width - width)/2 |
|
width: 2; height: parent.height * 0.23 |
|
radius: width |
|
smooth: true |
|
} |
|
} |
|
} |
hii I just need to know what if i have assets of these two, filling part and background how can i write the code then???
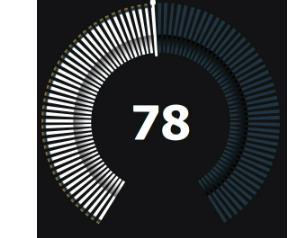