Created
September 26, 2019 01:08
-
-
Save Rowadz/30d39c73853445ab0f91fc9f240b2516 to your computer and use it in GitHub Desktop.
Monitoring binary logs via Nodejs
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const mysql = require('mysql'); | |
const MySQLEvents = require('@rodrigogs/mysql-events'); | |
const ora = require('ora'); // cool spinner | |
const spinner = ora({ | |
text: '🛸 Waiting for database events... 🛸', | |
color: 'blue', | |
spinner: 'dots2' | |
}); | |
const program = async () => { | |
const connection = mysql.createConnection({ | |
host: 'localhost', | |
user: 'root', | |
password: 'root' | |
}); | |
const instance = new MySQLEvents(connection, { | |
startAtEnd: true // to record only the new binary logs, if set to false or you didn'y provide it all the events will be console.logged after you start the app | |
}); | |
await instance.start(); | |
instance.addTrigger({ | |
name: 'monitoring all statments', | |
expression: 'TEST.*', // listen to TEST database !!! | |
statement: MySQLEvents.STATEMENTS.ALL, // you can choose only insert for example MySQLEvents.STATEMENTS.INSERT, but here we are choosing everything | |
onEvent: e => { | |
console.log(e); | |
spinner.succeed('👽 _EVENT_ 👽'); | |
spinner.start(); | |
} | |
}); | |
instance.on(MySQLEvents.EVENTS.CONNECTION_ERROR, console.error); | |
instance.on(MySQLEvents.EVENTS.ZONGJI_ERROR, console.error); | |
}; | |
program() | |
.then(spinner.start.bind(spinner)) | |
.catch(console.error); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
the logged data
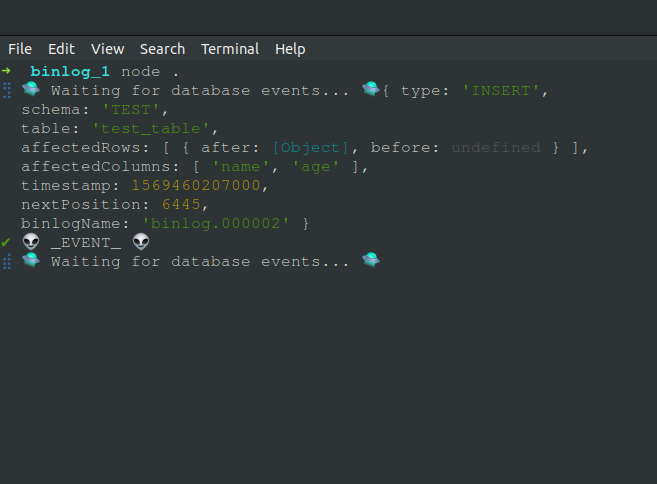