Last active
November 20, 2019 21:51
-
-
Save aal89/291614e39155f2d74ca8268be19e45d3 to your computer and use it in GitHub Desktop.
CC65 TGI NES demo that compiles (taken from https://github.com/cc65/cc65/blob/master/samples/tgidemo.c).
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <stdio.h> | |
#include <stdlib.h> | |
#include <cc65.h> | |
#include <conio.h> | |
#include <tgi.h> | |
#include <nes.h> | |
#include <joystick.h> | |
#define TGI_COLOR_BLACK 0x00 | |
#define TGI_COLOR_GREEN 0x01 | |
#define TGI_COLOR_VIOLET 0x02 | |
#define TGI_COLOR_WHITE 0x03 | |
#define TGI_COLOR_BLACK2 0x04 | |
#define TGI_COLOR_ORANGE 0x05 | |
#define TGI_COLOR_BLUE 0x06 | |
#define TGI_COLOR_WHITE2 0x07 | |
#define COLOR_BACK TGI_COLOR_BLACK | |
#define COLOR_FORE TGI_COLOR_WHITE | |
#define cgetcjoy while (!joy_read (JOY_1)) | |
/*****************************************************************************/ | |
/* Data */ | |
/*****************************************************************************/ | |
/* Driver stuff */ | |
static unsigned MaxX; | |
static unsigned MaxY; | |
static unsigned AspectRatio; | |
/*****************************************************************************/ | |
/* Code */ | |
/*****************************************************************************/ | |
static void CheckError (const char* S) | |
{ | |
unsigned char Error = tgi_geterror (); | |
if (Error != TGI_ERR_OK) { | |
cprintf ("%s: %d\n", S, Error); | |
if (doesclrscrafterexit ()) { | |
cgetcjoy; | |
} | |
exit (EXIT_FAILURE); | |
} | |
} | |
static void DoCircles (void) | |
{ | |
static const unsigned char Palette[2] = { TGI_COLOR_WHITE, TGI_COLOR_ORANGE }; | |
unsigned char I; | |
unsigned char Color = COLOR_FORE; | |
unsigned X = MaxX / 2; | |
unsigned Y = MaxY / 2; | |
tgi_setpalette (Palette); | |
while (!joy_read (JOY_1)) { | |
tgi_setcolor (COLOR_FORE); | |
tgi_line (0, 0, MaxX, MaxY); | |
tgi_line (0, MaxY, MaxX, 0); | |
tgi_setcolor (Color); | |
for (I = 10; I < 240; I += 10) { | |
tgi_ellipse (X, Y, I, tgi_imulround (I, AspectRatio)); | |
} | |
Color = Color == COLOR_FORE ? COLOR_BACK : COLOR_FORE; | |
} | |
cgetcjoy; | |
tgi_clear (); | |
} | |
static void DoCheckerboard (void) | |
{ | |
static const unsigned char Palette[2] = { TGI_COLOR_WHITE, TGI_COLOR_BLACK }; | |
unsigned X, Y; | |
unsigned char Color; | |
tgi_setpalette (Palette); | |
Color = COLOR_BACK; | |
while (1) { | |
for (Y = 0; Y <= MaxY; Y += 10) { | |
for (X = 0; X <= MaxX; X += 10) { | |
tgi_setcolor (Color); | |
tgi_bar (X, Y, X+9, Y+9); | |
Color = Color == COLOR_FORE ? COLOR_BACK : COLOR_FORE; | |
tgi_clear (); | |
return; | |
} | |
Color = Color == COLOR_FORE ? COLOR_BACK : COLOR_FORE; | |
} | |
Color = Color == COLOR_FORE ? COLOR_BACK : COLOR_FORE; | |
} | |
} | |
static void DoDiagram (void) | |
{ | |
static const unsigned char Palette[2] = { TGI_COLOR_WHITE, TGI_COLOR_BLACK }; | |
int XOrigin, YOrigin; | |
int Amp; | |
int X, Y; | |
unsigned I; | |
tgi_setpalette (Palette); | |
tgi_setcolor (COLOR_FORE); | |
/* Determine zero and aplitude */ | |
YOrigin = MaxY / 2; | |
XOrigin = 10; | |
Amp = (MaxY - 19) / 2; | |
/* Y axis */ | |
tgi_line (XOrigin, 10, XOrigin, MaxY-10); | |
tgi_line (XOrigin-2, 12, XOrigin, 10); | |
tgi_lineto (XOrigin+2, 12); | |
/* X axis */ | |
tgi_line (XOrigin, YOrigin, MaxX-10, YOrigin); | |
tgi_line (MaxX-12, YOrigin-2, MaxX-10, YOrigin); | |
tgi_lineto (MaxX-12, YOrigin+2); | |
/* Sine */ | |
tgi_gotoxy (XOrigin, YOrigin); | |
for (I = 0; I <= 360; I += 5) { | |
/* Calculate the next points */ | |
X = (int) (((long) (MaxX - 19) * I) / 360); | |
Y = (int) (((long) Amp * -_sin (I)) / 256); | |
/* Draw the line */ | |
tgi_lineto (XOrigin + X, YOrigin + Y); | |
} | |
cgetcjoy; | |
tgi_clear (); | |
} | |
static void DoLines (void) | |
{ | |
static const unsigned char Palette[2] = { TGI_COLOR_WHITE, TGI_COLOR_BLACK }; | |
unsigned X; | |
tgi_setpalette (Palette); | |
tgi_setcolor (COLOR_FORE); | |
for (X = 0; X <= MaxY; X += 10) { | |
tgi_line (0, 0, MaxY, X); | |
tgi_line (0, 0, X, MaxY); | |
tgi_line (MaxY, MaxY, 0, MaxY-X); | |
tgi_line (MaxY, MaxY, MaxY-X, 0); | |
} | |
cgetcjoy; | |
tgi_clear (); | |
} | |
int main (void) | |
{ | |
unsigned char Border; | |
joy_install (joy_static_stddrv); | |
/* Install the driver */ | |
tgi_install (tgi_static_stddrv); | |
CheckError ("tgi_install"); | |
tgi_init (); | |
CheckError ("tgi_init"); | |
tgi_clear (); | |
/* Get stuff from the driver */ | |
MaxX = tgi_getmaxx (); | |
MaxY = tgi_getmaxy (); | |
AspectRatio = tgi_getaspectratio (); | |
/* Set the palette, set the border color */ | |
Border = bordercolor (COLOR_BLACK); | |
/* Do graphics stuff */ | |
DoCircles (); | |
DoCheckerboard (); | |
DoDiagram (); | |
DoLines (); | |
#if DYN_DRV | |
/* Unload the driver */ | |
tgi_unload (); | |
#else | |
/* Uninstall the driver */ | |
tgi_uninstall (); | |
#endif | |
/* Reset the border */ | |
(void) bordercolor (Border); | |
/* Done */ | |
cprintf ("Done\n"); | |
joy_uninstall (); | |
return EXIT_SUCCESS; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Output:
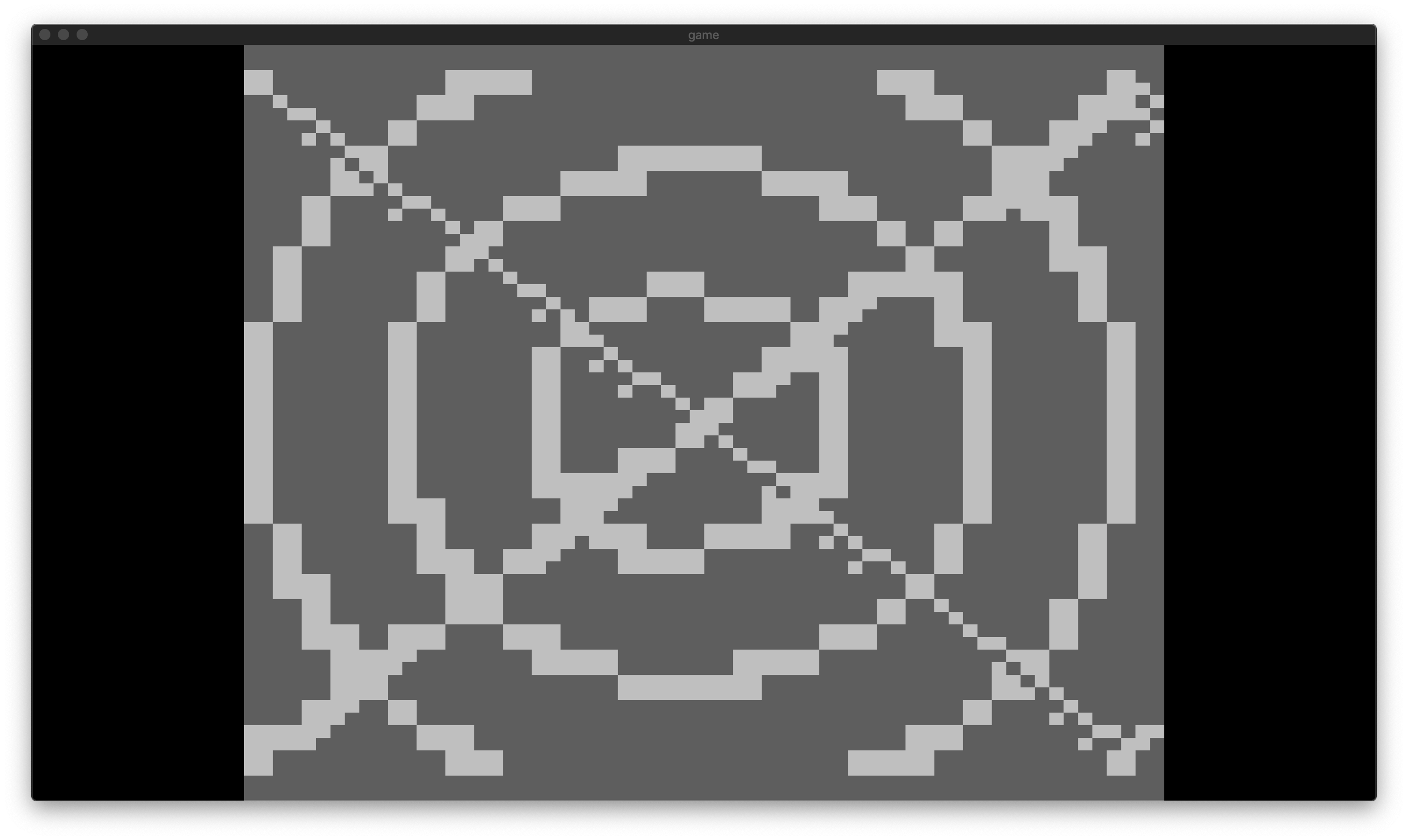