Last active
March 4, 2022 12:36
-
-
Save andremsantos/33781f39444efddbf619514104c55f7d to your computer and use it in GitHub Desktop.
Adding pagination to knex.js
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
module.exports = function(dbConfig) { | |
var knex = require('knex')(dbConfig); | |
var KnexQueryBuilder = require('knex/lib/query/builder'); | |
KnexQueryBuilder.prototype.paginate = function (per_page, current_page) { | |
var pagination = {}; | |
var per_page = per_page || 10; | |
var page = current_page || 1; | |
if (page < 1) page = 1; | |
var offset = (page - 1) * per_page; | |
return Promise.all([ | |
this.clone().count('* as count').first(), | |
this.offset(offset).limit(per_page) | |
]) | |
.then(([total, rows]) => { | |
var count = total.count; | |
var rows = rows; | |
pagination.total = count; | |
pagination.per_page = per_page; | |
pagination.offset = offset; | |
pagination.to = offset + rows.length; | |
pagination.last_page = Math.ceil(count / per_page); | |
pagination.current_page = page; | |
pagination.from = offset; | |
pagination.data = rows; | |
return pagination; | |
}); | |
}; | |
knex.queryBuilder = function () { | |
return new KnexQueryBuilder(knex.client); | |
}; | |
return knex; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Place it inside whenever folder name u want, in my case, under util folder
util / search.js
class Search {
constructor(query, queryString) {
this.query = query;
this.queryString = queryString;
this.tableName = this.query.and._single.table;
}
pagination(resPerPage) {
let { queryString, query, tableName } = this;
}
}
module.exports = SearchQuery;
on the other part:
// under the controller folder, create a file. "in my case, it is product.js"
const query = require("../config/database/database"); // this is came from knex configuration
const SearchQuery = require("../utils/searchQuery");
exports.getProducts = catchAsyncErrors(async (req, res, next) => {
const resPerPage = 5;
const search = new SearchQuery(query("products"), req.query)
.pagination(resPerPage);
const products = await search.query;
res.status(200).json({
success: true,
products,
});
});
this is working. kindly see screenshot below.
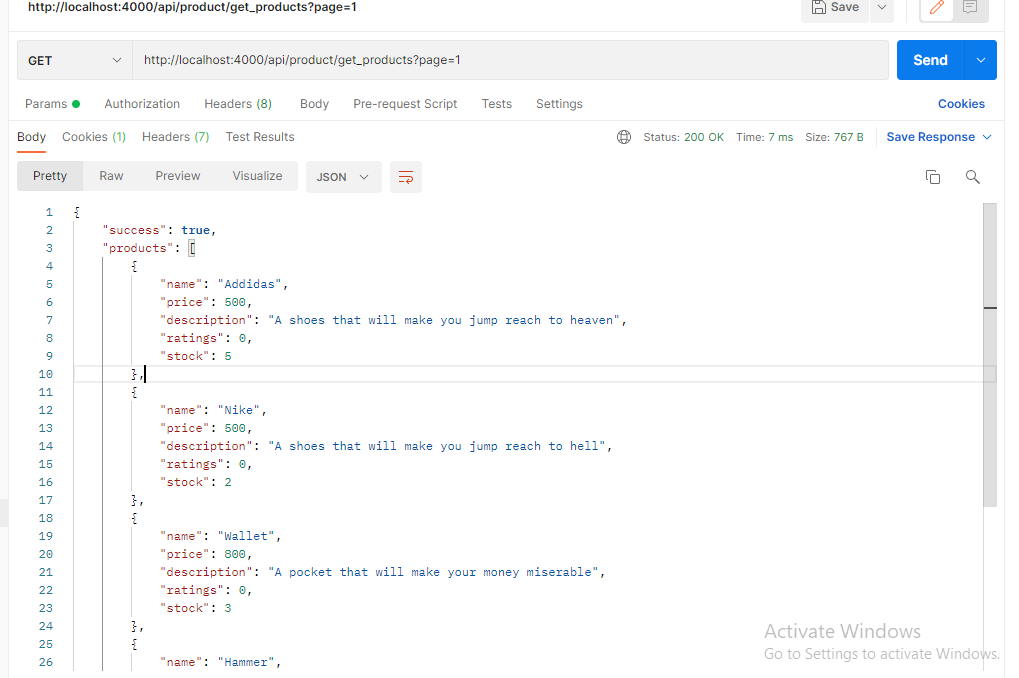
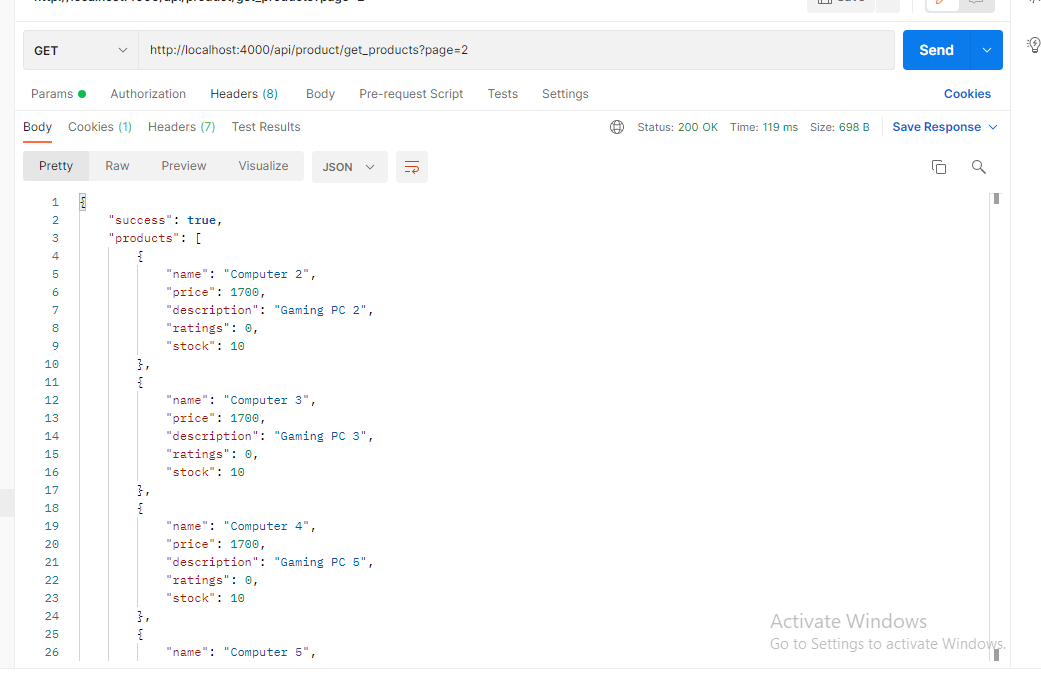