Last active
September 1, 2017 02:28
-
-
Save hassanshamim/f480ef19453cc9537479c66ba4f863d6 to your computer and use it in GitHub Desktop.
Cal helper files
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
print(""" September 2017 | |
Su Mo Tu We Th Fr Sa | |
1 2 | |
3 4 5 6 7 8 9 | |
10 11 12 13 14 15 16 | |
17 18 19 20 21 22 23 | |
24 25 26 27 28 29 30 | |
""") |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import sys | |
command_line_args = sys.argv[1:] # all but the first one | |
for arg in command_line_args: | |
print(arg) | |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import pytest # 'pip install pytest' in your virtual env | |
import subprocess # what we use to call the python file and the 'cal' command to get their output | |
MY_FILE = 'example_cal.py' # change to whatever yours is | |
def create_command(command, *args): # helper function | |
""" | |
Returns either | |
'python cal.py arg1 arg2' | |
or | |
'cal arg1 arg2' | |
to pass to the subprocess.getoutput function | |
Takes any number of args | |
i.e | |
>>> create_command('some_python_file.py' 8, 2017) | |
'python some_python_file 8 2017' | |
>>> create_command('cal', 2012) | |
'cal 2012' | |
""" | |
if command.endswith('py'): | |
command = 'python ' + command | |
args = [str(arg) for arg in args] | |
return ' '.join([command] + args) | |
def test_september_this_year(): | |
mine = create_command(MY_FILE, 9, 2017) | |
cals = create_command('cal', 9, 2017) | |
mine_output = subprocess.getoutput(mine) | |
cals_output = subprocess.getoutput(cals) | |
assert mine_output == cals_output | |
def test_feb_leap_year(): | |
mine = create_command(MY_FILE, 2, 2008) | |
cals = create_command('cal', 2, 2008) | |
mine_output = subprocess.getoutput(mine) | |
cals_output = subprocess.getoutput(cals) | |
assert mine_output == cals_output | |
def test_whole_year(): | |
mine = create_command(MY_FILE, 2001) | |
cals = create_command('cal', 2001) | |
mine_output = subprocess.getoutput(mine) | |
cals_output = subprocess.getoutput(cals) | |
assert mine_output == cals_output |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Getting command line arguments:
You can get the command line arguments passed into your python file
i.e.
python my_calendar 8 2011
will have the arguments '8' and '2011'These can be accessed via
sys.argv
. I've attached an example file that simply loops over any arguments passed in and prints them. Just copy the code up tocommand_line_args = ...
and you can use that if you wish.see: http://www.pythonforbeginners.com/system/python-sys-argv
Some example tests that compare the output of your code to the native 'cal' command.
Note: it's going to be finicky when it comes to spaces.
Testing
install pytest with:
source env/bin/activate
to activate your virtual envpip install pytest
Download the test_cal.py file and put it in the same folder as your cal.py (or whatever you called it). Edit
MY_FILE
to be the name of your calendar python file. In the above, i am pointing it toexample-cal.py
Run the tests with:
pytest -xvv test_cal.py
the
-x
means stop after first failing testthe
vv
means very verbose, show me the full difference.RED is a faling test
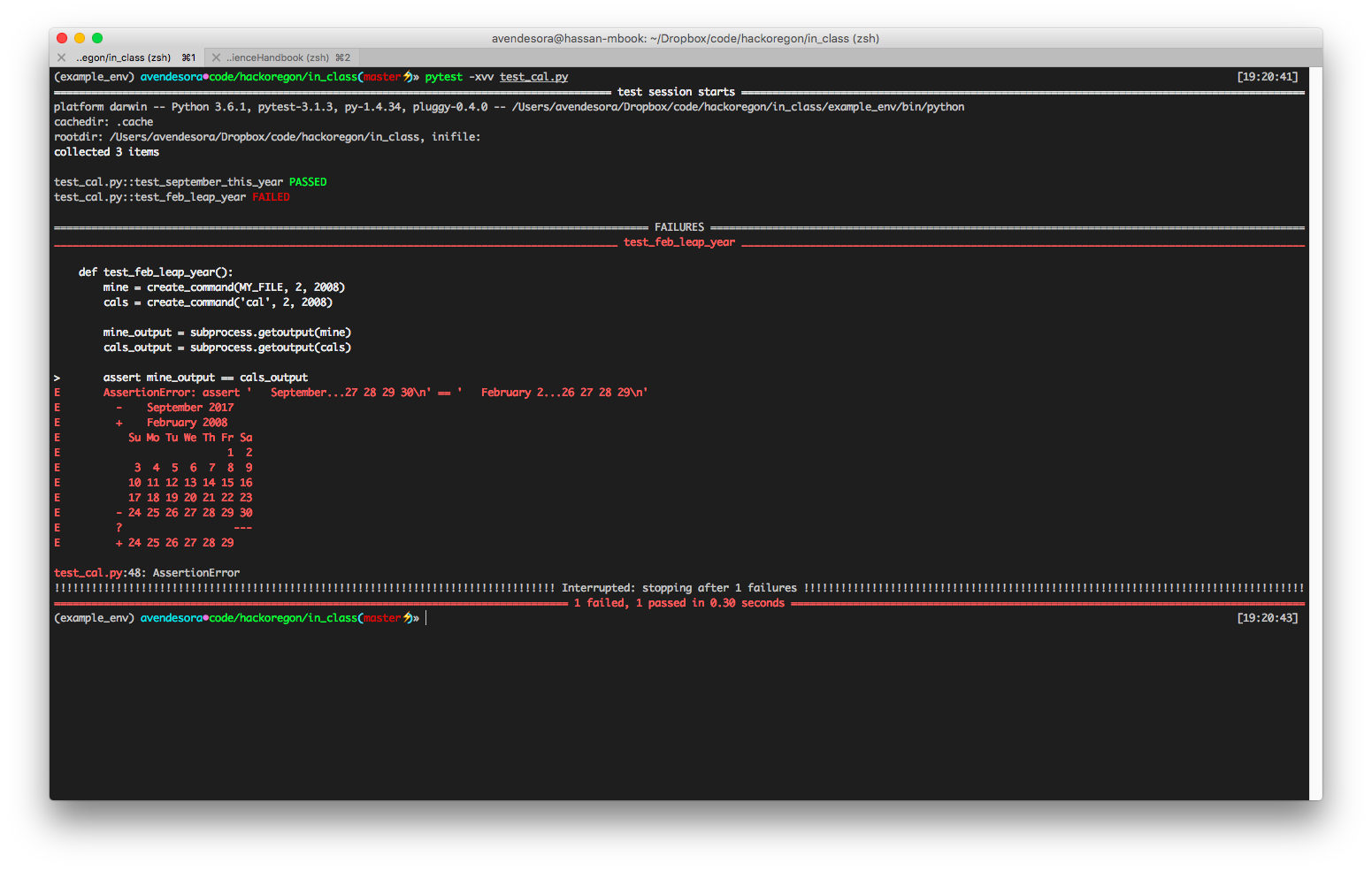
GREEN is a test that passed.
should look something like